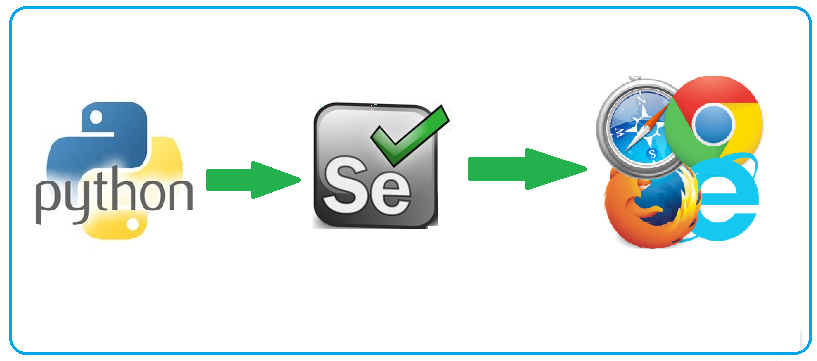
In today’s fast-paced digital landscape, ensuring the quality and reliability of web applications is paramount. As websites become more complex and dynamic, manual testing alone is no longer sufficient to guarantee flawless performance across various platforms and browsers. This is where automated testing tools like Selenium come into play, empowering developers and testers to streamline their testing processes and detect issues early on.
Selenium, an open-source testing framework, has emerged as a popular choice for automating web browsers. Its versatility, coupled with the flexibility of Python, makes it a compelling combination for web testing. In this article, we’ll explore how Python and Selenium synergize to create robust and efficient automated testing solutions.
Table of Contents
ToggleWhy Selenium with Python
Python’s simplicity and readability have made it one of the most widely adopted programming languages, and its integration with Selenium brings several advantages:
Ease of Use
Python’s clean syntax and extensive libraries simplify the process of writing and maintaining test scripts. Testers can focus more on the logic of their tests rather than dealing with intricate syntax, resulting in faster development cycles.
Abundance of Libraries
Python boasts a rich ecosystem of libraries and frameworks that complement Selenium, offering additional functionalities for tasks such as data manipulation, test reporting, and integration with other tools.
Cross-Platform Support
Python’s cross-platform compatibility ensures that test scripts written on one operating system can seamlessly run on others, reducing compatibility issues and enhancing scalability.
Community Support
Both Python and Selenium have vibrant communities that actively contribute to their development. This means ample documentation, tutorials, and forums where developers can seek assistance and share best practices.
Getting Started with Selenium and Python
To begin harnessing the power of Selenium with Python, you’ll need to set up your development environment. Here’s a step-by-step guide:
Install Python
If Python is not already installed on your system, download and install it from the official website (https://www.python.org/). Make sure to add Python to your system’s PATH during installation.
Install Selenium
You can install Selenium using Python’s package manager, pip, by running the following command in your terminal or command prompt:
pip install selenium
Download WebDriver
WebDriver is a crucial component of Selenium that allows interaction with web browsers. Depending on the browser you intend to automate , download the respective WebDriver executable and ensure it’s accessible in your system’s PATH.
Write Your First Test
With Selenium and Python installed, you’re ready to write your first test script. Here’s a simple example that opens a browser, navigates to a webpage, and verifies its title:
from selenium import webdriver
# Initialize the browser driver
driver = webdriver.Chrome() # Change this to the appropriate WebDriver
# Open the webpage
driver.get("https://example.com")
# Verify the title
assert "Example Domain" in driver.title
# Close the browser
driver.quit()
Explore Advanced Features
Selenium offers a plethora of features for handling complex scenarios, such as interacting with web elements, handling pop-ups, executing JavaScript, and performing actions like mouse clicks and keyboard inputs. Dive deeper into Selenium’s documentation to leverage these capabilities effectively.
Best Practices for Selenium Testing with Python
While Selenium simplifies automated testing, adopting best practices is crucial for maximizing its potential:
Use Page Object Model
Implement the POM design pattern to enhance the maintainability and reusability of your test code. This involves creating separate classes for each webpage, encapsulating their elements and functionalities.
Parameterize Your Tests
Parameterizing tests allows for greater coverage with minimal code duplication. Leverage Python’s testing frameworks like pytest or unittest to incorporate data-driven testing and manage test suites efficiently.
Handle Waits Appropriately
Synchronization is vital in automated testing to ensure that tests wait for elements to be present or become interactive. Use Selenium’s WebDriverWait along with Expected Conditions to handle waits effectively and avoid flakiness in your tests.
Implement Logging and Reporting
Integrate logging and reporting mechanisms into your test suite to track test execution, identify failures, and generate comprehensive reports. Python’s logging module combined with reporting tools like Allure or pytest-html can aid in this aspect.
Regular Maintenance
Web applications evolve over time, so should your test suite. Regularly review and update your test scripts to accommodate changes in the application’s UI or functionality, ensuring that your tests remain accurate and reliable.
Conclusion
By combining the power of Selenium with the simplicity of Python, developers and testers can build robust automated testing frameworks capable of validating web applications across different browsers and platforms efficiently. Whether you’re a seasoned automation engineer or a novice tester, mastering Selenium with Python opens up a world of possibilities for accelerating your testing workflows and delivering high-quality software products. So, dive in, explore, and empower your testing endeavors with this dynamic duo.